Introduction to Android Security
Building secure Android apps isn't just a recommendation; it's a necessity in today's digital age where security threats loom large. At the heart of Android security lies the principle of least privilege: this means giving an app only those permissions it absolutely needs to work, nothing more. It's key to prevent misuse of sensitive data and protect against malicious attacks. Android's security model is built around three core areas: the app sandbox, app signing, and permissions. When you develop an Android app, you work within a sandbox that isolates your app's data and code execution from other apps. This minimizes the risk of data leaks or breaches. App signing ensures that the app comes from a legitimate source and hasn't been tampered with. Lastly, the permissions system asks users to grant permissions for an app to access certain data or features on their device. It's crucial to handle this responsibly, only requesting what's absolutely necessary for your app to function. Remember, a secure app not only earns user trust but is also a step forward in maintaining a safer digital environment.
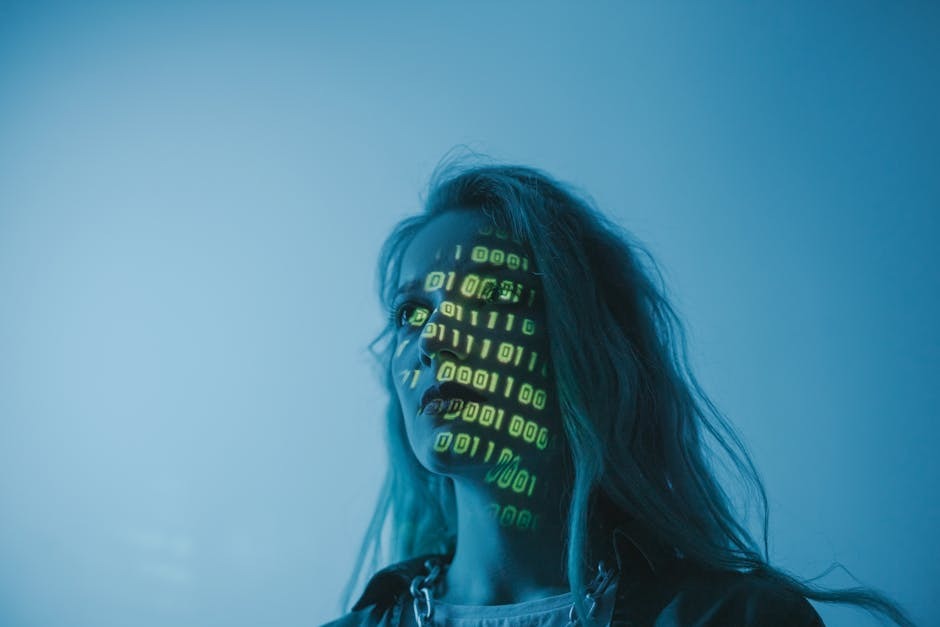
Understanding Programming Best Practices for Secure Apps
In app development, security isn’t a feature; it's a necessity. When it comes to building secure Android apps, there are several programming best practices you need to follow. First, always validate input from users. This means checking data entered into your app to ensure it’s what you expect. If you skip this step, attackers could sneak harmful data into your system. Next, update third-party libraries often. Developers use these libraries for added functionality, but they can have vulnerabilities. Staying updated means you reduce risk. Also, don’t store sensitive information like passwords directly in your app. Instead, use encryption or tokenization to protect this data. And, of course, use secure communication protocols such as HTTPS to safeguard data during transfer. Keeping these practices in mind will help you build apps that not only function well but also protect your users’ information. Remember, a secure app builds trust, and trust keeps users coming back.
The Importance of Data Encryption in Android Apps
When you're building an app, you want to make sure no one can sneak in and steal your user's data. That's where data encryption comes in. Think of it as turning your user's information into a secret code. Even if someone bad gets their hands on it, they can't understand it. For Android apps, this is critical. Your users trust you with their private information. If you're not using encryption, you're risking that trust. Plus, Google and legal rules are pretty clear about keeping user data safe. Not doing so can land you in big trouble. Simple as that, encryption helps keep your app and your users secure. Every Android developer needs to use it, no excuses.
Implementing Secure User Authentication
When building Android apps, getting user authentication right is crucial. It's the line of first defense against unauthorized access. Here's how to do it: Use strong encryption methods for passwords. Don't just store passwords as is. Encrypt them using robust algorithms like SHA-256. Better yet, consider using a tried-and-tested authentication library, so you don't reinvent the wheel. Implement two-factor authentication (2FA). It adds another layer of security. Even if a password is compromised, an attacker still needs the second factor—usually a code sent to the user's phone—to gain access. Keep an eye on session management. Make sure session tokens expire after a reasonable period. This limits the damage if a session token is stolen. Remember, securing your app is not just about protecting data; it's about safeguarding your users' trust.
Guarding Against SQL Injection and Other Common Vulnerabilities
To make your Android app tough against attacks, especially SQL injection, you need to be smart about your code. SQL injection, where attackers sneak malicious code into your database through poorly secured input fields, is a big deal. It's like leaving your door unlocked for thieves. But you can lock the door tight. Start by always using prepared statements or parameterized queries when you deal with database operations. This isn't just a fancy term; it's your safety net, ensuring that incoming data is treated as data, not executable code.
And don't forget about validating and sanitizing user input. Think of it as a bouncer at a club, deciding what gets in and what stays out. If an input looks sketchy or doesn't fit the rules (e.g., an email field containing anything other than an email), block it or clean it up.
Besides guarding against SQL injection, focus on other vulnerabilities too. Keep your code updated. It’s like getting a vaccine to protect against new diseases. Use the latest security features and patches available for the Android platform. Also, encrypt sensitive information. If your app handles passwords, credit card numbers, or any private data, encryption turns it into a secret code. Even if someone sneaks past your defenses, they can't understand or misuse this encrypted data.
Remember, securing your app is like gearing up for a battle. You're not just building functionality; you're fortifying against threats. Stay vigilant and make security your top priority.
Using HTTPS and SSL to Protect Data in Transit
When you're building an Android app, you gotta keep it safe. That means making sure no bad guys can sneak a peek at your users' data while it's moving from the app to the server and back. Enter HTTPS and SSL—your digital bodyguards. HTTPS, which stands for HyperText Transfer Protocol Secure, is like a secure version of HTTP. It uses SSL, short for Secure Sockets Layer, to create a secure tunnel for data transmission. Think of SSL as a protective bubble that wraps around your data, keeping it safe from hackers. When you use HTTPS and SSL in your app, you're making sure that all data sent over the Internet is encrypted. This means even if someone intercepts it, they can't understand it. It’s like speaking in a secret code that only you and the intended recipient can decipher. To set this up, you’ll need an SSL certificate for your server. This certificate is like a digital ID that proves your server is legit. Once you have it, make sure your app connects to your server using HTTPS, not HTTP. This is crucial for protecting sensitive information like passwords, credit card numbers, and personal details. Remember, building a secure app isn’t just a nice-to-have; it’s a must-do to protect your users. So, always use HTTPS and SSL. It's a simple step, but it makes a big difference in keeping your app safe from prying eyes.
Key Android Security Features Developers Should Utilize
Key Android security features are like armor for your app. Let's break down the essentials. First up, Android App Sandbox. Every app gets its own little playground, away from others. No app messes with another; it's a rule. Next, we've got Permissions. Apps must ask for your okay before using your data or features on your device. No surprises. Network Security Configuration is another big player. It lets developers shape how their app trusts the web, ensuring safe communication. Don't forget about SafetyNet. It checks devices for security threats, keeping the bad stuff out. Also, Google Play Protect scans apps on the Play Store, providing a safety net before you even download. Lastly, Storage Encryption keeps your data locked up tight, only accessible to those with the key. Utilize these tools, and you're well on your way to building a fortress of an app.
How to Securely Store Sensitive Information in Android Apps
Storing sensitive information in Android apps needs careful handling. Rule number one is never to keep sensitive data directly on a device if you can avoid it. But, when you must, use Android's built-in security features to your advantage. Start with SharedPreferences for storing user settings or preferences. Though convenient, encrypt the data first because SharedPreferences are not secure by default. For encryption, Android Keystore system is your go-to. It creates and manages cryptographic keys in a secure manner. Also, consider leveraging SQLCipher, an open-source extension of SQLite, for encrypting the entire database. Remember, storing passwords, credit card information, or personal details demands stringent measures. Always hash passwords with a salt before storing them. If you're transmitting data, use HTTPS to protect it in transit. Don't store keys or sensitive information in your source code. Instead, use secure servers or services for key management. In short, be smart, use Android’s security features wisely, and always encrypt sensitive information before storing it.
Regular Updates and Maintenance: Keeping Your App Secure
Keeping your app secure doesn't end once it's built and launched. The digital world's constant evolution means new threats pop up regularly. That's why updating and maintaining your app is crucial. Think of it as taking your car for regular check-ups; just like your car, your app needs this attention to perform its best and stay safe. With each update, you have the chance to fix bugs, patch security holes, and even add new features that users will love. Remember, hackers love finding and exploiting outdated software. So, if you slack on updates, you're practically inviting them in. Ensure your app goes through regular check-ups to keep it running smoothly and securely. This isn't just a one-time deal; it's an ongoing commitment to your users' safety and your app's success.
Conclusion: Integrating Best Practices for Long-Term App Security
Wrapping up, making your Android app tough as nails isn't a one-and-done deal. It's about weaving together the right practices into the very fabric of your app. Remember, security isn't just a fancy feature; it's the backbone of trust between your app and your users. By embedding best practices into your development process—like writing clean, understandable code, keeping third-party libraries up to date, and rigorously testing for vulnerabilities—you're not just avoiding trouble today. You're setting up your app for a future where it stands strong against threats. So, keep learning, keep improving, and make security your top priority from day one. Your users will thank you, and your app will be better for it.